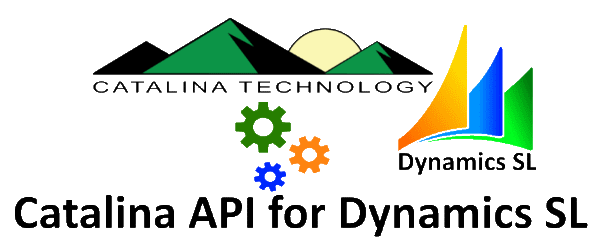
How to Add a Web Reference to a CTAPI Web Service in Visual Studio
Often, I get questions on how to start with the Catalina API for Dynamics SL. We are going to be doing a series of posts that walk through how to use our API for Dynamics SL.
This post is focused on how to create a web reference in Visual Studio to consume our SOAP based web services. This is pretty simple and we will build on it in posts to come.
Under Solution Explorer
- Right Click on “References”
- Select “Service References”
On the “Add Service Reference” screen
- Click the “Advanced” button in the bottom left corner.
On the “Service Reference Settings” screen
- Click the “Add Web Reference” button in the bottom left corner.
On the “Add Web Reference” screen
- Enter the URL to the catalina SOAP service you want to call.
eg: http://localhost/ctDynamicsSL/vendorMaintenance.asmx - You can get a list of all service available on the index page via a web browser.
eg: http://localhost/ctDynamicsSL/
- Click the arrow button to the left of the URL to get the web service listing.
- After discovery, enter a name to refer to this web reference in your client in the “Web Reference name” textbox. In our example we will use directory.filename.
eg: ctDynamicsSL.vendorMaintenance
- Click the “Add Reference” button.
- The new reference will now show up under Web References listing in solution explorer.
In your project code file, add the code to instantiate the service object
- Private variable to store our object reference:
private ctDynamicsSL.vendorMaintenance.vendorMaintenance myVendorsServiceValue = null;
- Add a property that will dynamically create the web service object with all required SOAP Header values:
- The SOAP Header (ctDynamicsSLHeader) is required for all CTAPI web services.
- In our example, we set the values from the app.config file using ConfigurationManager, but that is easily customizable.
public ctDynamicsSL.vendorMaintenance.vendorMaintenance myVendorsService { get { if (myVendorsServiceValue == null) { ctDynamicsSL.vendorMaintenance.ctDynamicsSLHeader Header = new ctDynamicsSL.vendorMaintenance.ctDynamicsSLHeader(); Header.siteID = System.Configuration.ConfigurationManager.AppSettings["SITEID"]; Header.cpnyID = System.Configuration.ConfigurationManager.AppSettings["CPNYID"]; Header.licenseKey = System.Configuration.ConfigurationManager.AppSettings["LICENSEKEY"]; Header.licenseName = System.Configuration.ConfigurationManager.AppSettings["LICENSENAME"]; Header.licenseExpiration = System.Configuration.ConfigurationManager.AppSettings["LICENSEEXPIRATION"]; Header.siteKey = System.Configuration.ConfigurationManager.AppSettings["SITEKEY"]; Header.softwareName = "CTAPI"; myVendorsServiceValue = new ctDynamicsSL.vendorMaintenance.vendorMaintenance(); myVendorsServiceValue.ctDynamicsSLHeaderValue = Header; myVendorsServiceValue.Timeout = System.Threading.Timeout.Infinite; } return myVendorsServiceValue; } set { myVendorsServiceValue = value; } }
Screenshot of the Visual Studio IDE

Example of how the code looks in the Visual Studio IDE